In the last article, we explored the fundamental aspects of Ethereum, and we gained a good understanding of the platform and its potential for building decentralized applications.
In this article, we will delve into a practical aspect of Ethereum development by creating a smart contract on the platform. Specifically, we will build a lottery smart contract that allows users to purchase tickets and have a chance to win a prize. We will also deploy this smart contract on an Ethereum testnet. By the end of this article, you should have a solid understanding of how to develop and deploy smart contracts on Ethereum.
Understanding the Lottery Smart Contract
A lottery system is a game of chance where participants purchase tickets for a chance to win a prize. In a decentralized lottery system, a smart contract is used to handle the ticket-purchasing process and randomly select a winner.
The smart contract is a self-executing program that is stored on the Ethereum blockchain. It is written in Solidity, a programming language specifically designed for creating smart contracts.
The lottery smart contract is implemented using a few key features of Solidity, such as variables, functions, and control structures. The contract will keep track of the number of tickets sold, the amount of ETH raised, and the addresses of the participants. The contract will also take care of checking that the buyer sends the correct amount of ETH and recording their address. Finally, the contract will contain a function to randomly select a winner from the list of participants.
Building the Lottery Smart Contract
Now that we have a basic understanding of the lottery system and how smart contracts work, let’s dive into building our lottery smart contract. We will be using Solidity version 0.8.0 for this project.
First, we need to set up our development environment. For this part, we can use Remix, a web-based IDE for developing Solidity smart contracts, or we can install a local development environment like Ganache or Truffle.
After choosing a coding platform, we can write the smart contract we will use for this article:
pragma solidity ^0.8.0; contract Lottery { address manager; address payable[] public players; address payable public winner; constructor() { manager=msg.sender; } receive() external payable { require(msg.value==0.01 ether,“Pay 0.01 Ether to participate in the lottery!”); players.push(payable(msg.sender)); } function Get_Balance() public view returns(uint) { require(msg.sender==manager,”This function is callable by The manager only.“); return address(this).balance; } function random() internal view returns(uint) { return uint(keccak256(abi.encodePacked(block.difficulty,block.timestamp,players.length))); } function Pick_Winner() public { require(msg.sender==manager,”This functino is callable by the manager only.“); require(players.length>=3,”The number of participants is still less than 3“); uint rand=random(); uint winners_index=rand%players.length; winner=players[winners_index]; winner.transfer(Get_Balance()); players=new address payable[](0); } function All_Players() public view returns(address payable[] memory) { return players; } } |
The code above is an example of a simple lottery smart contract. Let’s break it down:
- The contract is called “Lottery”.
- The “manager” is the address of the person who deploys the contract and is responsible for picking the winner.
- The “players” array is a list of all the addresses of the participants in the lottery.
- The “winner” variable is the address of the winner of the lottery.
- The constructor function is called when the contract is deployed and sets the manager to be the address of the person who deployed the contract.
- The “receive” function is called when someone sends Ether to the contract. The function checks that the sender has sent exactly 0.01 Ether and adds their address to the “players” array.
- The “Get_Balance” function can be called by the manager to get the current balance of the contract.
- The “random” function generates a random number using the current block difficulty, timestamp, and the length of the “players” array. It is worth noting that the method used to generate a random number is not considered entirely secure for use in a production environment as it could potentially be manipulated by miners. It is mainly used for educational purposes. In a real-world scenario, you would need to implement a more secure method for generating a random number, such as using an oracle or a verifiable random function (VRF).
- The “Pick_Winner” function can be called by the manager to pick a winner. The function checks that there are at least three players and then generates a random number to select the winner from the “players” array. The winner receives the entire balance of the contract, and the “players” array is reset to an empty array.
- The “All_Players” function returns the array of all players.
After writing the code, we will test the smart contract on a testnet, like Goerli, to ensure that it functions as expected. Testing is an essential step before deploying a smart contract on the main Ethereum network to avoid errors and potential financial losses.
MetaMask Wallet
MetaMask is a popular Ethereum wallet that allows users to interact with Ethereum-based applications, also known as dApps, directly from their web browser. It is a browser extension that can be installed on Chrome, Firefox, Brave, and other web browsers.
MetaMask is one of the easiest ways to deploy smart contracts to a testnet, such as Goerli, and interact with them. To use MetaMask, you first need to install it on your web browser. Once installed, you can create a new wallet or import an existing one.
To set up MetaMask wallet, follow these steps:
- Install the MetaMask extension on your web browser.
- Create a new MetaMask wallet or import an existing one.
- Connect MetaMask to a test network like Goerli by clicking on the network dropdown and selecting the desired network.
- Fund your wallet with test Ether from a faucet or exchange that supports testnet tokens.
Once you have set up MetaMask, you can connect it to Remix IDE to deploy your smart contract to the Goerli testnet. To connect MetaMask to Remix IDE, follow these steps:
- Open Remix IDE in your web browser.
- Click on the “Deploy and run transactions” tab.
- Click on the “Environment” dropdown and select “Injected Provider – MetaMask”.
- If your MetaMask wallet is unlocked and connected to the Goerli network, you should see your account address in the “Account” dropdown.
- Once you are connected, you can deploy your smart contract to the Goerli testnet and interact with it using Remix IDE.
Using MetaMask with Remix IDE makes it easy to deploy and test your smart contracts on a test network, without the need for additional infrastructure or tools.
For the next section, we will create three different accounts -We will name them Account1, Account2, and Account3– in our MetaMask wallet, fund them with Goerli Ether using a Goerli faucet, and use them to test our smart contract once we deploy it on Goerli testnet.
Deploying the Smart Contract to Goerli Testnet
Once you have written and tested the smart contract locally, the next step is to deploy it on the Goerli testnet. Deploying the smart contract on the Goerli testnet enables you to test it in an environment that closely simulates the Ethereum mainnet without having to spend real ether.
To deploy the smart contract to the Goerli testnet, we will be using Remix IDE and the MetaMask wallet. To get started, first, we need to connect our wallet to Remix IDE, following the steps outlined in the previous section.
After successfully connecting your wallet, it’s time to deploy the smart contract. To do this, navigate to the “Deploy & run transactions” tab in Remix IDE, select the “Injected Provider – MetaMask” environment, and deploy the smart contract using your account. Keep in mind that you need to have some Goerli Ether in your account to complete this step.
Once you have deployed the smart contract using your MetaMask wallet, you will be prompted to confirm the transaction. Here is an example of what the confirmation pop-up will look like:
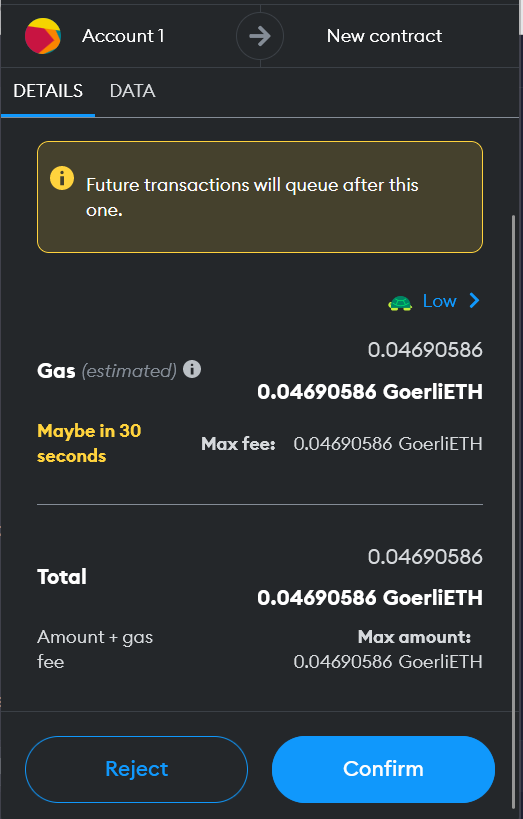
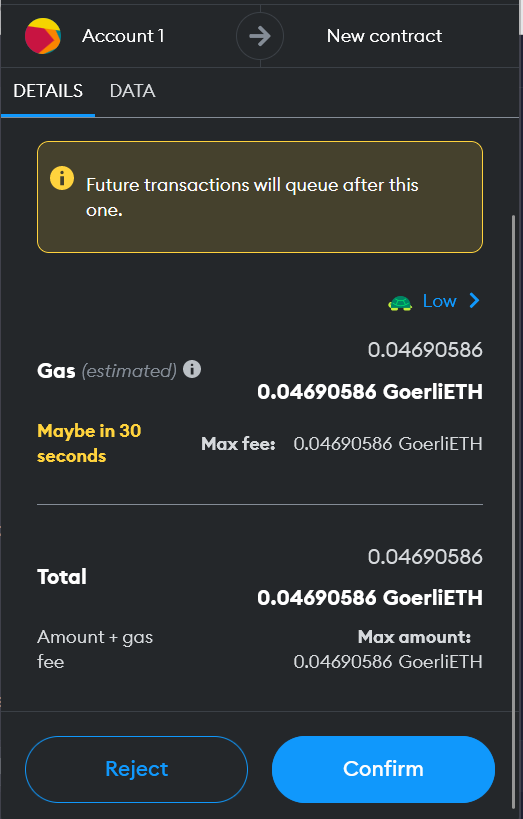
After confirming the transaction, you need to wait for some time for the transaction to be completed and for the contract to be live on the Goerli testnet. Keep in mind that the values might differ, as the gas price is always changing.
Once the smart contract has been successfully deployed on the Goerli testnet, you can find it on “goerli.etherscan.io” by searching for its address on the website. You can find the contract address in the “Deployed Contracts” section of the “Deploy & run transactions” tab. On the etherscan page of the contract, you can also access additional information, such as the “Transaction Hash”, “Block Number”, “Timestamp”, “Gas Price”, and much more.
In this example, Account1 is the owner of the smart contract and therefore the manager.
As the smart contract has been deployed and our wallet is still connected to Remix IDE, we can participate in the lottery using all our 3 accounts (including the manager) directly from the IDE. To do this, we can switch between the different accounts in the wallet and make transactions to the lottery smart contract. Here’s an example of making a transaction to the smart contract using Account2:
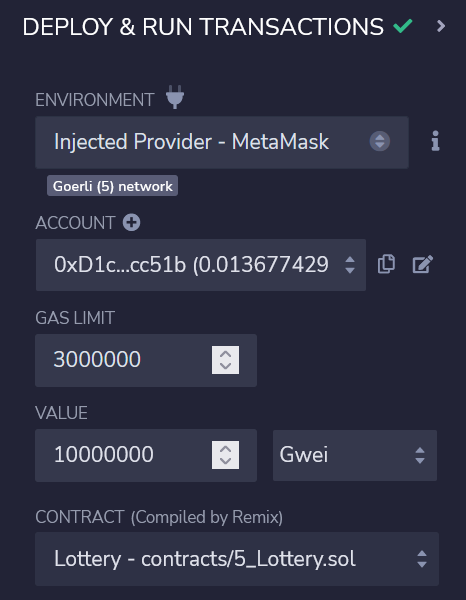
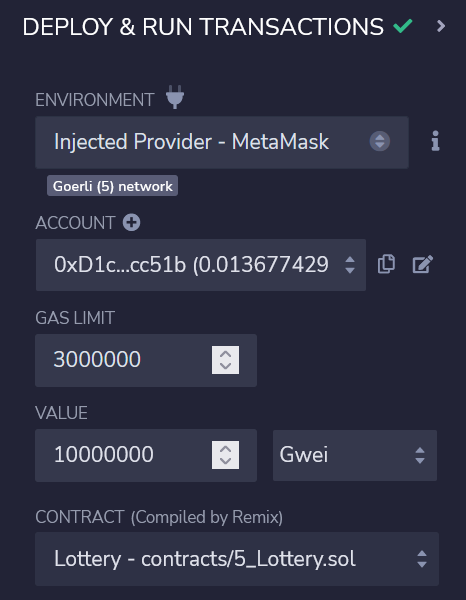
It is worth mentioning that 10000000 Gwei is equal to 0.001 Ether.
After participating in the lottery using all three accounts, we can switch back to using Account1 as the manager and call the different functions of the smart contract. Here’s an example of calling a function on the smart contract using Account1:
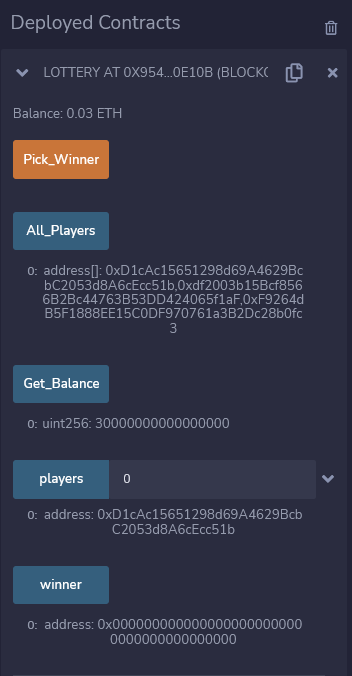
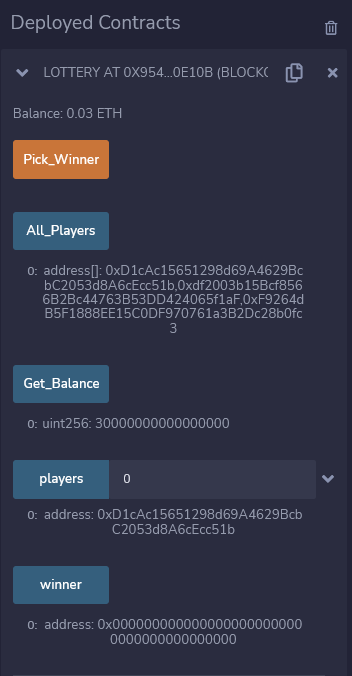
Now that we’ve tested all the functions and verified that they’re working properly, it’s time to randomly choose a winner from the participants using the “Pick_Winner” function. Note that only the manager (Account1) can call this function. Here’s an example of calling the “Pick_Winner” function to choose a winner:
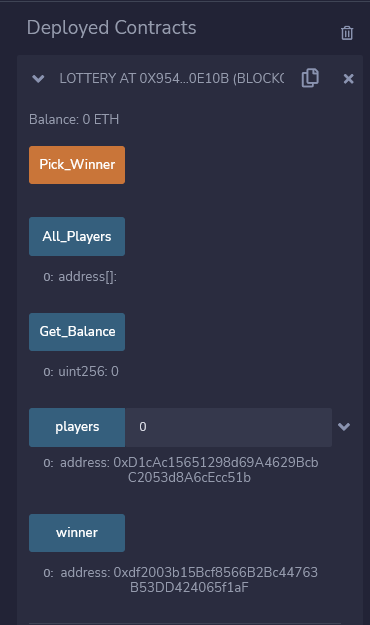
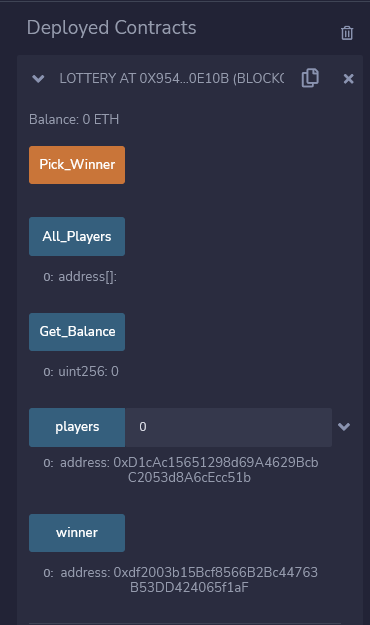
After calling the “Pick_Winner” function, the array is reset, the contract balance is zero, and Account3 is declared the winner. The prize money of 0.3 Ether is automatically transferred to Account3.
In summary, deploying a smart contract on the Goerli testnet using Remix IDE and MetaMask wallet is a straightforward process that allows developers to test their contracts in a simulated environment without having to spend real ether. Once deployed, the contract can be accessed and interacted with using Remix IDE and MetaMask wallet. Additionally, information about the deployed contract can be found on etherscan.io, including the contract address, transaction hash, block number, timestamp, and gas price. By testing all the functions of the smart contract and randomly choosing a winner using the “Pick_Winner” function, developers can ensure that their contract is working properly and ready for deployment on the Ethereum mainnet.
Final Words
In this article, we have successfully built and deployed a simple lottery smart contract on the Ethereum blockchain. We have learned about the lottery system, the Solidity programming language, and the deployment process on the Goerli testnet using Remix IDE and MetaMask wallet.
We have also tested the smart contract on the Goerli testnet and verified its functionality. This project is just a starting point, and there is a lot more that can be done with smart contracts on the Ethereum blockchain.
In the future, building decentralized applications (dApps) using smart contracts will become more prevalent. Smart contracts provide a secure and transparent way of executing code without the need for intermediaries. The use cases for smart contracts are limitless, and they have the potential to revolutionize the way we conduct transactions and interact with each other on the internet.
Overall, this project has provided a solid foundation for understanding smart contracts and their deployment process. We encourage you to continue exploring the world of blockchain and smart contracts and discover new ways to use this technology.